Vue js Find full Capitalized Words from String: To find words that are fully capitalized (all capital letters) in a string using Vue.js, you can split the string into an array of words using the split()
method. Then, you can use a regular expression to test each word in the array and see if it consists entirely of capital letters. If it does, you can push it to a new array of capitalized words.
What is the most efficient way to identify capitalized words within a string using Vue.js?
This is a Vue.js app that finds fully capitalized words in a text input using regular expressions. It then filters out any words that have lowercase letters and stores the fully capitalized words in an array.
Sure! This is a code snippet written in Vue.js, a popular JavaScript framework used for building user interfaces.
The code creates a Vue app using the Vue.createApp()
method and defines a data
object with two properties: text
and fullyCapitalizedWords
.
The text
property is intended to hold the user’s input text, while the fullyCapitalizedWords
array will store any fully capitalized words found in that input text.
The methods
object defines a single method called findFullyCapitalizedWords()
. This method uses a regular expression to search for fully capitalized words in the text
property using the match()
method.
The /\b[A-Z]+\b/g
regular expression matches any sequence of one or more uppercase letters (A to Z) that are surrounded by word boundaries (i.e., whitespace or punctuation).
The || []
syntax ensures that an empty array is returned if there are no matches.
Finally, the method filters out any words in the resulting array that have lowercase letters using the filter()
method and the /[a-z]/
regular expression.
The resulting fullyCapitalizedWords
array is then updated with the filtered results.
Vue Js Find Capitalized Words in String Example
<div id="app">
<label for="text">Enter text:</label>
<textarea id="text" v-model="text" @input="findFullyCapitalizedWords"></textarea>
<p>Fully capitalized words:</p>
<ul>
<li v-for="(word, index) in fullyCapitalizedWords" :key="index">{{ word }}</li>
</ul>
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
text: '',
fullyCapitalizedWords: []
};
},
methods: {
findFullyCapitalizedWords() {
const fullyCapitalizedWords = this.text.match(/\b[A-Z]+\b/g) || [];
this.fullyCapitalizedWords = fullyCapitalizedWords.filter(word => !word.match(/[a-z]/));
}
}
});
app.mount('#app')
</script>
Output of Vue Js find word that are fully captalized
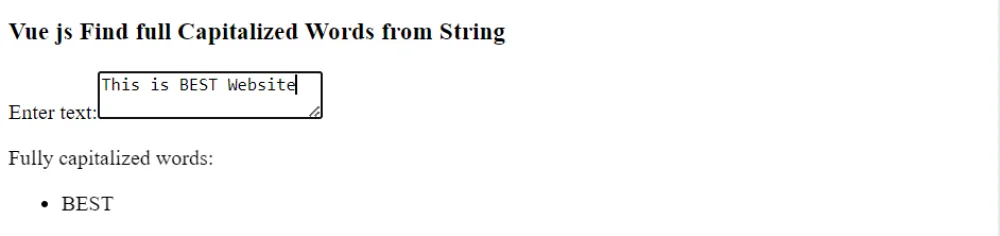